VB.NET - How To Display Data From The Selected DataGridView Row IntoTextBoxes Using VB.NET
In This VB.NET Tutorial We Will See How To Displaying The Selected DataGridView Row Values To TextBox Using VB.NET Programming Language.
Project Source Code:
Imports System.Data.DataTable
Public Class Selected_Row_From_DataGridView_To_TextBoxes
' Create a new datatable
Dim table As New DataTable("Table")
Private Sub Selected_Row_From_DataGridView_To_TextBoxes_Load(sender As Object, e As EventArgs) Handles MyBase.Load
' Add columns to your datatable,
' with the name of the columns and their type
table.Columns.Add("Id", Type.GetType("System.Int32"))
table.Columns.Add("First Name", Type.GetType("System.String"))
table.Columns.Add("Last Name", Type.GetType("System.String"))
table.Columns.Add("Age", Type.GetType("System.Int32"))
' Add rows to the datatable with some data
table.Rows.Add(1, "XXXX", "YYYYY", 21)
table.Rows.Add(2, "SSDD", "hGSQ", 33)
table.Rows.Add(3, "fgfgd", "jgfdd", 53)
table.Rows.Add(4, "cvfghyghj", "sdrgtyh", 19)
table.Rows.Add(5, "hghfd", "ghjgdf", 36)
table.Rows.Add(6, "cvvdfgh", "juyrfdvc", 63)
' load datatable data to datagridview DataGridView1.DataSource = table End Sub
' datagridview cell click event
Private Sub DataGridView1_CellClick(sender As Object, e As DataGridViewCellEventArgs) Handles DataGridView1.CellClick
Dim index As Integer
' get the index of the selected datagridview row
index = e.RowIndex
Dim selectedRow As DataGridViewRow
selectedRow = DataGridView1.Rows(index)
' now show data from the selected row to textboxes
TextBoxID.Text = selectedRow.Cells(0).Value.ToString()
TextBoxFN.Text = selectedRow.Cells(1).Value.ToString()
TextBoxLN.Text = selectedRow.Cells(2).Value.ToString()
TextBoxAGE.Text = selectedRow.Cells(3).Value.ToString()
End Sub
End Class
///////////////OUTPUT:
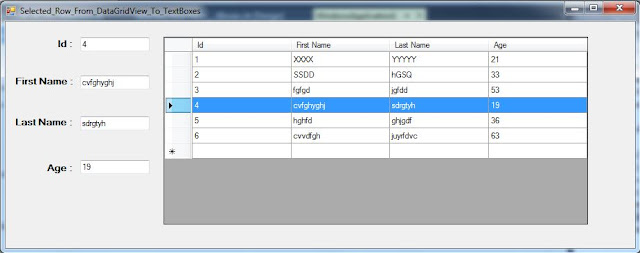
Selected Row From DataGridView To TextBoxes Using VB.NET
Download Projects Source Code
3 comments
commentsI from brazil, nice code :)
ReplyI'm from Brazil, very much with your code. **
ReplyVery helpful. Thank you!
Reply